There is no better way to learn than to get our hands dirty and implement the code. Let’s get started:
- We will start in Cloud Shell by creating the terraform directory.
- Per Terraform’s best practices, we will create a file called main.tf.
- We will specify the Terraform provider as hashicorp/google:
terraform {
required_providers {
google = {
source = “hashicorp/google”
version = “4.51.0”
}
}
}
provider “google” {
project = “wmarusiak-terraform-project”
region = “europe-west4”
zone = “europe-west4-c”
}
resource “google_compute_network” “vpc_network” {
name = “terraform-network”
} - This section of the code deploys a new project (if one doesn’t exist) and creates a VPC network named terraform-network.
- Before we can use the code, we need to initiate the Terraform provider. To do so, we must use the terraform init command:
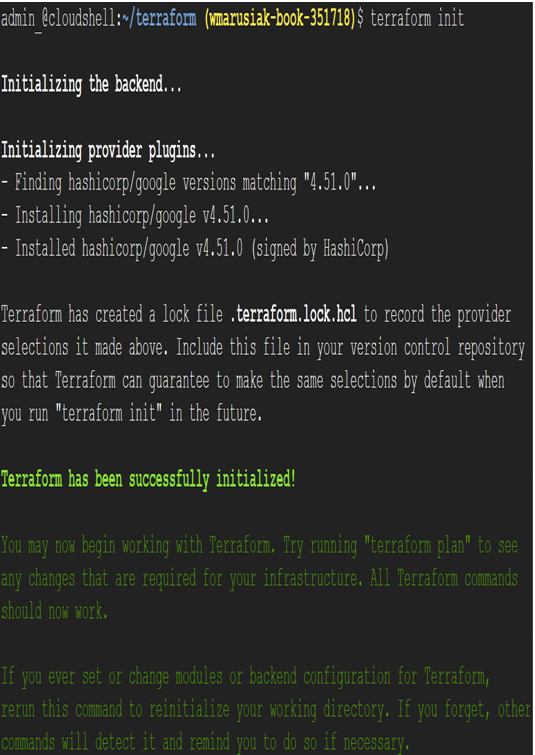
Figure 7.36 – Successfully initializing the Terraform provider
- Before we implement the code, it is a good practice to validate our code and check which resources the code creates. We can use the terraform apply command to do this, which checks if we have permission to create specified resources and gives us an overview of the resources to be created. To validate the code, we can use the terraform validate command. To show changes that are required by the current configuration, we need to use the terraform plan command:
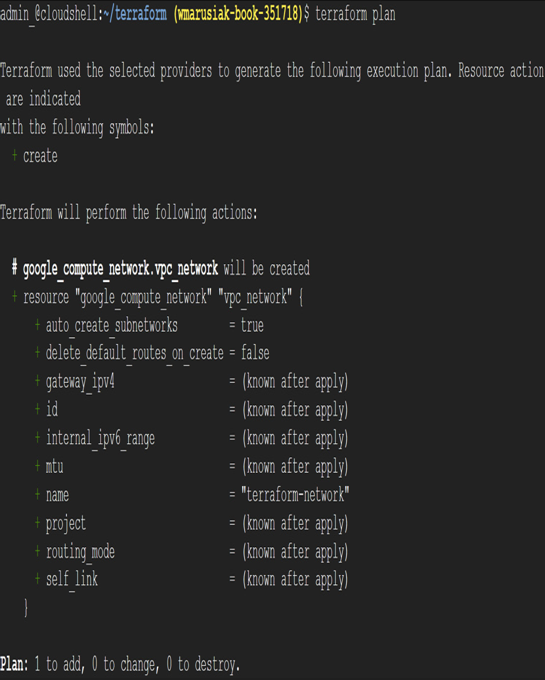
Figure 7.37 – Terraform validates and shows the execution plan
- Finally, we can use the terraform apply command to create the resources. As a final check, we will be asked if we want to create specified resources; we need to type yes to execute the code.
- After a moment, the code will be implemented, and we can use the newly created resources:
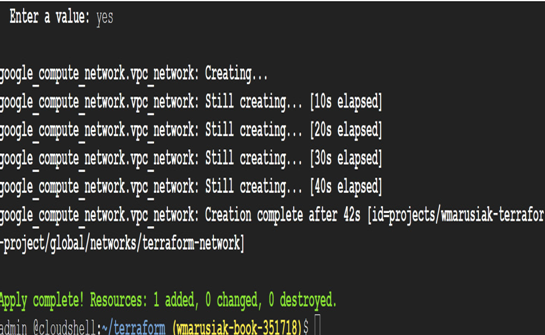
Figure 7.38 – Terraform code execution completed
- After successfully implementing code with Terraform, we can delete the resources that were created using the terraform destroy command.
- After a moment, the resources will be deleted:
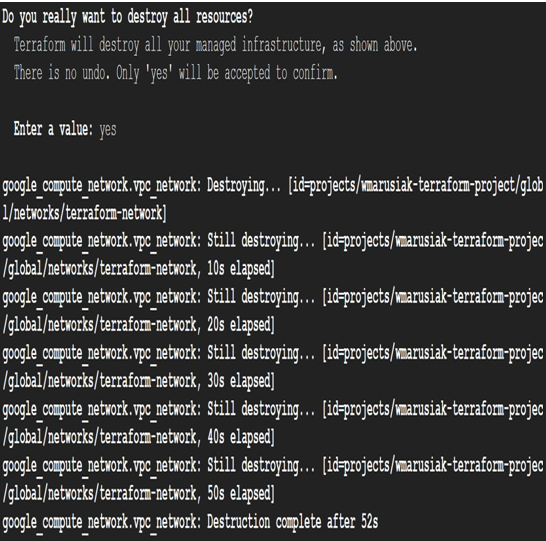
Figure 7.39 – Resource deletion is finished
As this example has shown, using Terraform in Google Cloud is straightforward.
Terraform best practices
Google Cloud has published Terraform’s best practices at https://cloud.google.com/docs/terraform/best-practices-for-terraform. These best practices cover topics such as the following:
- Module structure
- Naming conventions
- Using variables
- And many more
Similar cloud-agnostic Hashicorp-provided best practices for using Terraform are available at – https://developer.hashicorp.com/terraform/cloud-docs/recommended-practices
With the recent updates, Google Cloud introduced a possibility to view Terraform Code when we create a new virtual machine. You can view the Terraform code snippet after clicking the on Equivalent Code button in the Google Compute Engine section.
In the next section, we will build on the skills we’ve learned in this section and implement a sample template in the Google Cloud project.