Now that you’ve learned what Cloud Functions is, I’d like to show you how to implement a sample Cloud Function.
We will guide you through optical character recognition (OCR) on Google Cloud Platform with Cloud Functions.
Our use case is as follows:
- An image with text is uploaded to Cloud Storage.
- A triggered Cloud Function utilizes the Google Cloud Vision API to extract the text and identify the source language.
- The text is queued for translation by publishing a message to a Pub/Sub topic.
- A Cloud Function employs the Translation API to translate the text and stores the result in the translation queue.
- Another Cloud Function saves the translated text from the translation queue to Cloud Storage.
- The translated results are available in Cloud Storage as individual text files for each translation.
We need to download the samples first; we will use Golang as the programming language. Source files can be downloaded from – https://github.com/GoogleCloudPlatform/golang-samples. Before working with the OCR function sample, we recommend enabling the Cloud Translation API and the Cloud Vision API. If they are not enabled, your function will throw errors, and the process will not be completed.
Let’s start with deploying the function:
- We need to create a Cloud Storage bucket. Create your own bucket with unique name – please refer to documentation on bucket naming under following link: https://cloud.google.com/storage/docs/buckets. We will use the following code:
gsutil mb gs://wojciech_image_ocr_bucket - We also need to create a second bucket to store the results:
gsutil mb gs://wojciech_image_ocr_bucket_results - We must create a Pub/Sub topic to publish the finished translation results. We can do so with the following code: gcloud pubsub topics create YOUR_TOPIC_NAME. We used the following command to create it:
gcloud pubsub topics create wojciech_translate_topic - Creating a second Pub/Sub topic to publish translation results is necessary. We can use the following code to do so:
gcloud pubsub topics create wojciech_translate_topic_results - Next, we will clone the Google Cloud GitHub repository with some Python sample code:
git clone https://github.com/GoogleCloudPlatform/golang-samples - From the repository, we need to go to the golang-samples/functions/ocr/app/ file to be able to deploy the desired Cloud Function.
- We recommend reviewing the included go files to review the code and understand it in more detail. Please change the values of your storage buckets and Pub/Sub topic names.
- We will deploy the first function to process images. We will use the following command:
gcloud functions deploy ocr-extract-go –runtime go119 –trigger-bucket wojciech_image_ocr_bucket –entry-point ProcessImage –set-env-vars “^:^GCP_PROJECT=wmarusiak-book-351718:TRANSLATE_TOPIC=wojciech_translate_topic:RESULT_TOPIC=wojciech_translate_topic_results:TO_LANG=es,en,fr,ja” - After deploying the first Cloud Function, we must deploy the second one to translate the text. We can use the following code snippet:
gcloud functions deploy ocr-translate-go –runtime go119 –trigger-topic wojciech_translate_topic –entry-point TranslateText –set-env-vars “GCP_PROJECT=wmarusiak-book-351718,RESULT_TOPIC=wojciech_translate_topic_results” - The last part of the complete solution is a third Cloud Function that saves results to Cloud Storage. We will use the following snippet of code to do so:
gcloud functions deploy ocr-save-go –runtime go119 –trigger-topic wojciech_translate_topic_results –entry-point SaveResult –set-env-vars “GCP_PROJECT=wmarusiak-book-351718,RESULT_BUCKET=wojciech_image_ocr_bucket_results” - We are now free to upload any image containing text. It will be processed first, then translated and saved into our Cloud Storage bucket.
- We uploaded four sample images that we downloaded from the Internet that contain some text. We can see many entries in the ocr-extract-go Cloud Function’s logs. Some Cloud Function log entries show us the detected language in the image and the other extracted text:
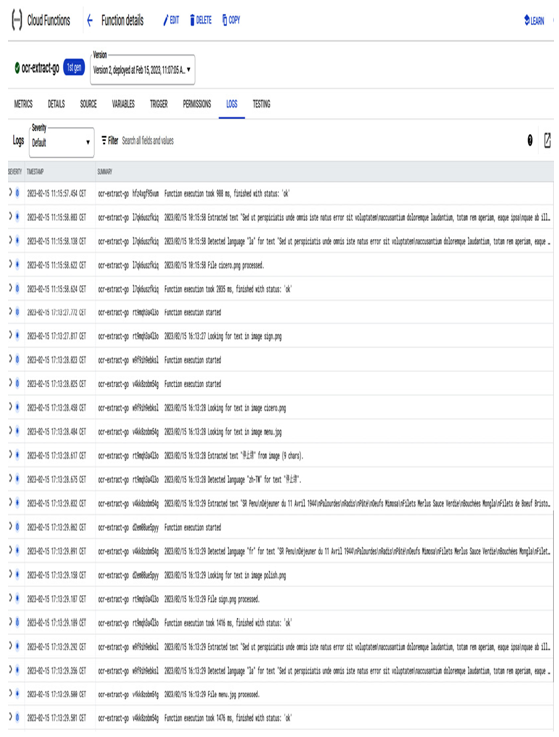
Figure 7.22 – Cloud Function logs from the ocr-extract-go function
13. ocr-translate-go translates detected text in the previous function:
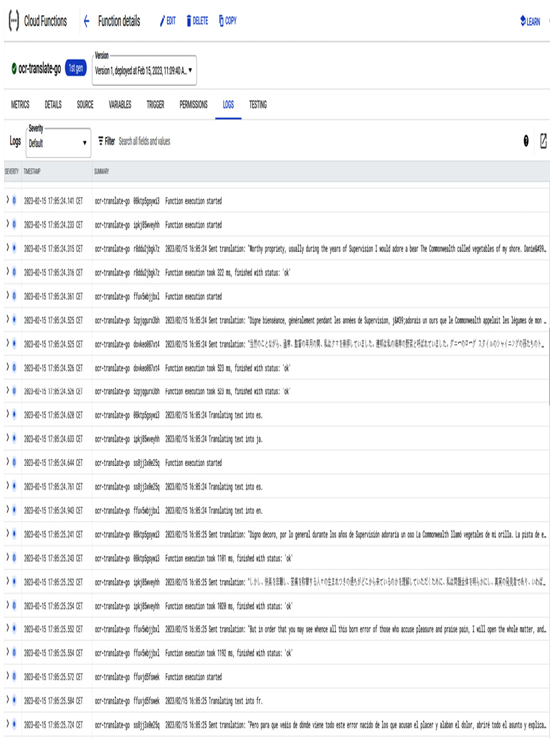
Figure 7.23 – Cloud Function logs from the ocr-translate-go function
14. Finally, ocr-save-go saves the translated text into the Cloud Storage bucket:
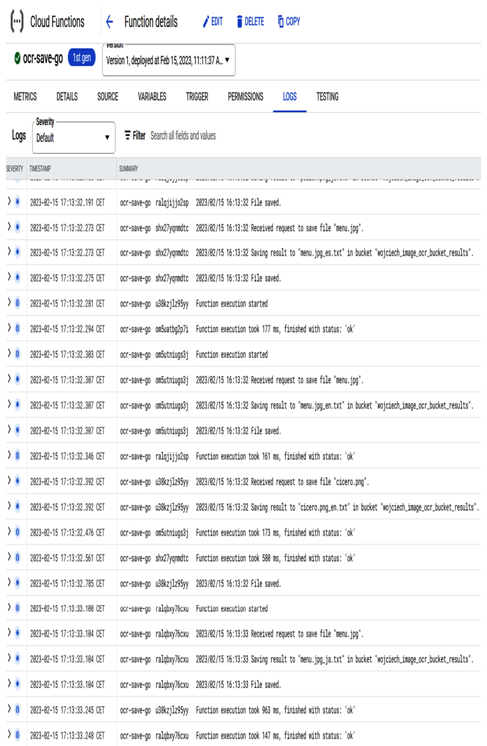
Figure 7.24 – Cloud Function logs from the ocr-save-go function
15. If we go to the Cloud Storage bucket, we’ll see the saved translated files:
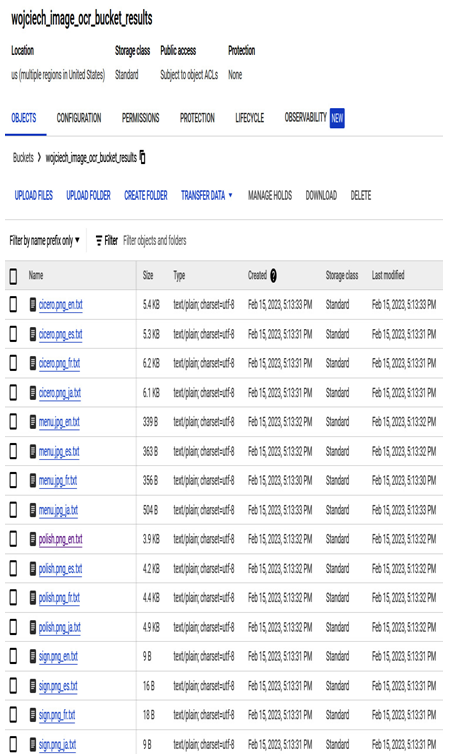
Figure 7.25 – Translated images saved in the Cloud Storage bucket
16. We can view the content directly from the Cloud Storage bucket by clicking Download next to the file, as shown in the following screenshot:
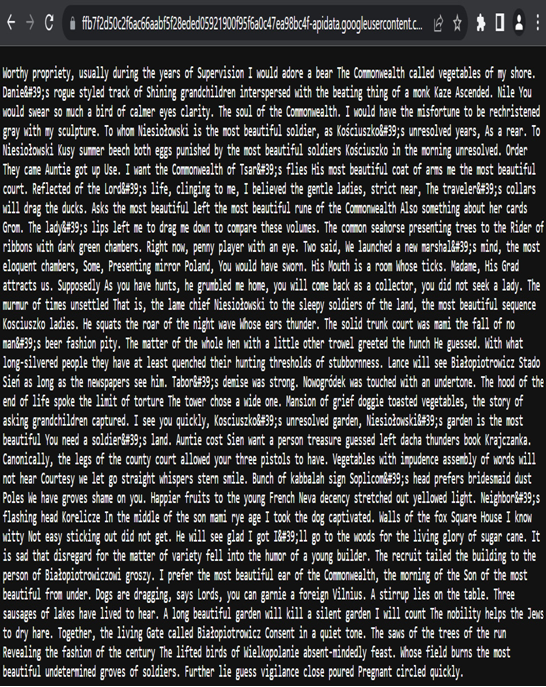
Figure 7.26 – Translated text from Polish to English stored in the Cloud Storage bucket
Cloud Functions is a powerful and fast way to code, deploy, and use advanced features. We encourage you to try out and deploy Cloud Functions to understand the process of using them better.
At the time of writing, Google Cloud Free Tier offers a generous number of free resources we can use. Cloud Functions offers the following with its free tier:
- 2 million invocations per month (this includes both background and HTTP invocations)
- 400,000 GB-seconds, 200,000 GHz-seconds of compute time
- 5 GB network egress per month
Google Cloud has comprehensive tutorials that you can try to deploy. Go to https://cloud.google.com/functions/docs/tutorials to follow one.
Now that we’ve covered the serverless products in Google Cloud, we will learn about IaC.